The append
and appendChild
Javascript methods are both used to — as their names imply — insert items at the end of the elements or node object they’re attached to. As much as they both can be used interchangeably, there are a few subtle differences between the two methods.
- The
append
method is used to insert either Node objects or DOMStrings (basically a string or text) into the DOM, while theappendChild
method can only be used to insert Node objects into the DOM.
For example, with the append
method, you can do:
let div = document.createElement("div")
//insert a DOM node
let p = document.createElement("p");
div.append(p);
console.log(div) //<div><p></p></div>
//insert a DOMString
div.append(‘<p>Hello</p>’)
console.log(div) //<div><p>Hello</p></div>
With the appendChild
method,
let div = document.createElement("div")
//insert a DOM node
let p = document.createElement("p");
div.appendChild(p);
console.log(div) //<div><p></p></div>
//insert a DOMString
div.appendChild(‘<p>Hello</p>’) //This results in an Uncaught TypeError: Failed to execute 'appendChild' on 'Node'
- The
append
method can insert multiple items at once, but theappendChild
method can’t.
div.append(child, childTwo); // Works fine
div.appendChild(child, childTwo,); // Works fine, but adds the first element and ignores the rest
- According to caniuse, the
append
method is not supported in IE.
In summary, appendChild
can be used interchangeably with append, but not vice versa.
Blog Credits: Linda Ikechukwu
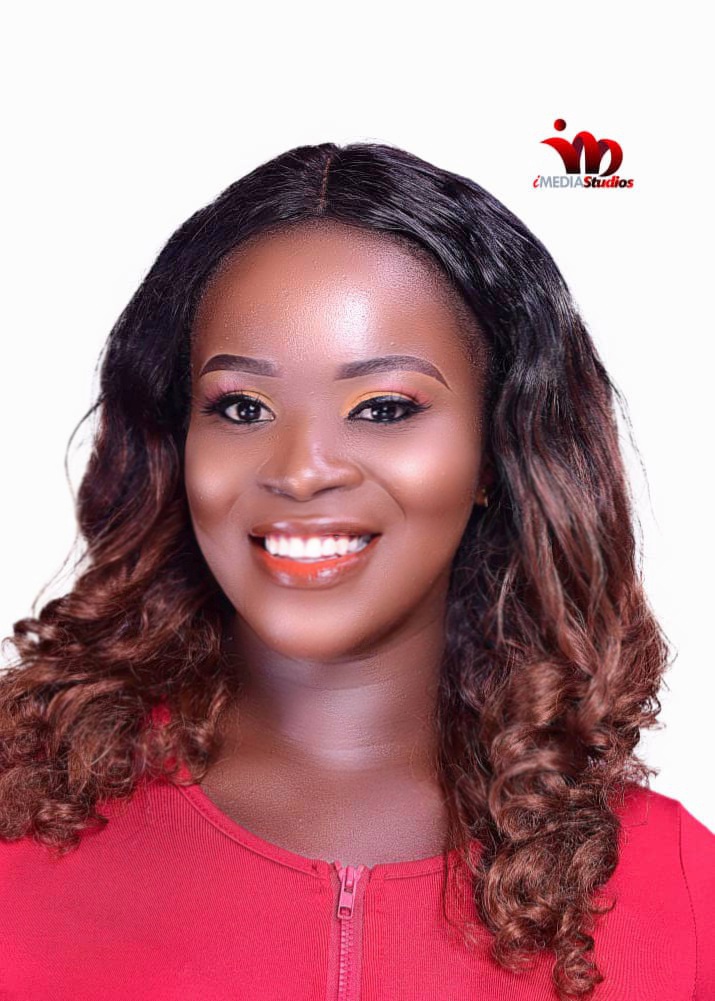
Comments are closed.