Hooks were released by the React team in v16.8 as functions that let you “hook into” React state and lifecycle features from function components to let you use React without classes. React currently has a number of pre-defined hooks, but also lets you write yours in form of custom hooks.
A custom Hook is simply a JavaScript function used to extract component logic into reusable functions. React specifies that custom hook names must be prefixed with ‘use’ to ensure that they can be automatically checked for violations of the rules of Hooks.
For example, one common functionality found in most applications is updating input values with values entered by the user on the onChange event.
Take this below for example:
import React, {useState} from 'react';
const App = () => {
const [email, setEmail] = useState("");
const [password, setPassword] = useState("");
return (
<div className="App">
<h1>Demo App</h1>
<input type="text" placeholder="Email" onChange= {e => setEmail(e.target.value)} />
<input type="password" placeholder="Password" onChange= {e => setPassword(e.target.value)} />
<button onClick={(e) => handleSubmit(e)}>Submit</button>
</div>
);
}
export default App;
We could extract this functionality into a custom hook, so we won’t have to write several corresponding onChange function within each input (especially, if we had more inputs on the page).
When writing a custom React hook, it’s advisable to have a separate folder within your project folder named utils or libs or whatever name you wish, specifically for reusable functions. Individual files for individual custom hooks may then be placed within that folder.
So in src/utils/useInputValue.js, we’ll have this:
import { useState } from "react";
const useInputValue = initialValue => {
const [value, setValue] = useState(initialValue);
return {
value,
onChange: e => {
setValue(e.target.value || e.target.innerText);
}
};
}
export default useInputValue;
The useInputValue
hook simply takes in an initial value, sets it as the value for the value variable received from useState
, and returns an object containing the value and an onChange
function to update that value.
This way, we can use the useInputValue
hook in App.js like so:
import React from 'react';
import useInputValue from './useInputValue';
const App = () => {
const email = useInputValue("");
const password = useInputValue("");
return (
<div className="App">
<h1>Demo App</h1>
<input type="text" placeholder="Email" {...email} />
<input type="password" placeholder="Password" {...password} />
<button onClick={(e) => handleSubmit(e)}>Submit</button>
</div>
);
}
export default App;
The {…email} syntax spreads the return value of useInputValue, which is equivalent to value=”somevalue” onChange={e=> setValue(e.target.value || e.target.innerText
)}
Blog Credits: Linda Ikechukwu
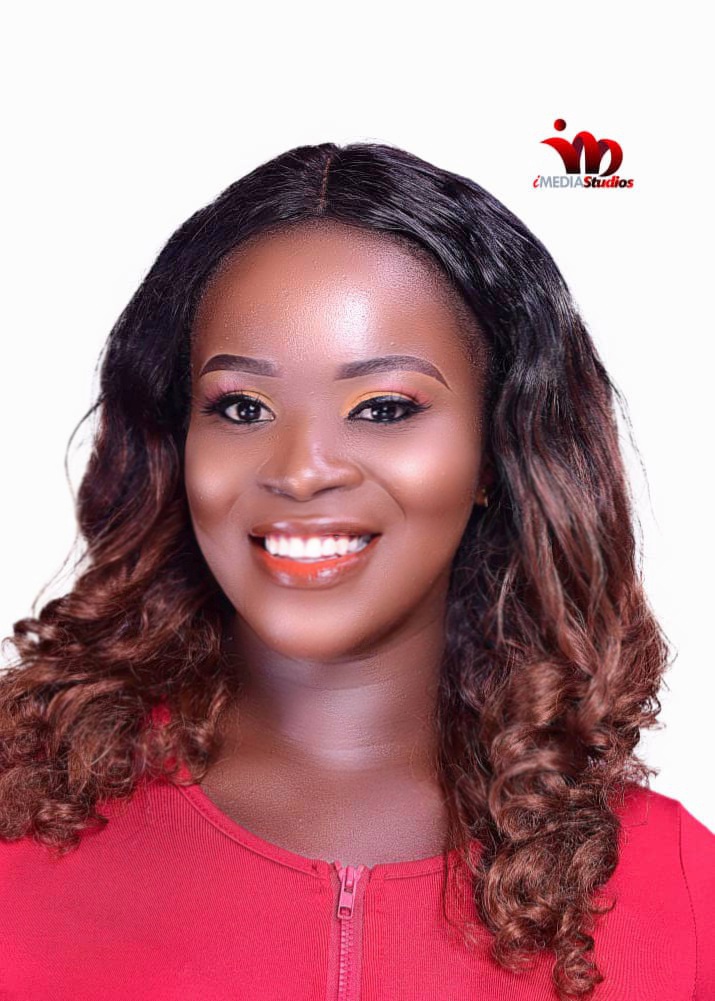
Comments are closed.