If you’ve been writing React for a while, then you know what JSX is. JSX is the custom syntax extension of JavaScript. It allows us to write HTML markup code with React.
One shock beginners to React always face is finding out that they can’t use the beloved for loop (or its variations, ie ‘for of
’, ‘for in
’) within the return statements of React components. This always produces an error: “Syntax error: Unexpected token
”.
const SampleComponent = () =>{
//I am a for loop. Can I stay here? YES!!!
return(
<div>
//I am a for loop. Can I stay here? NO!!
</div>
)
}
You can’t use the for
loop within the return statements of React components for the same reasons you can’t use for loops within the return
statement of a function in vanilla JavaScript. Variable declarations or complex statements are not allowed within return
statements. And, to use a for loop, you need to define a variable with let
or var
:
for(let i=0; i<5; i++){
....
}
The map method is the surest way to loop inside React JSX if the element to be iterated is an array:
const SampleComponent = () => {
const animals = [
{ id: 1, animal: "Dog" },
{ id: 2, animal: "Pig" },
{ id: 3, animal: "Cat" },
{ id: 4, animal: "Bird" },
{ id: 5, animal: "Snake" }
];
return (
<div>
{animals.map(item => (
<p key={item.id}>{item.animal}</p>
))}
</div>
);
};
If the element to be iterated is not an array, then you can either convert it to an array using Array.from()
or carry out the required looping technique outside the return
statement of the React component.
Blog Credits: Linda Ikechukwu
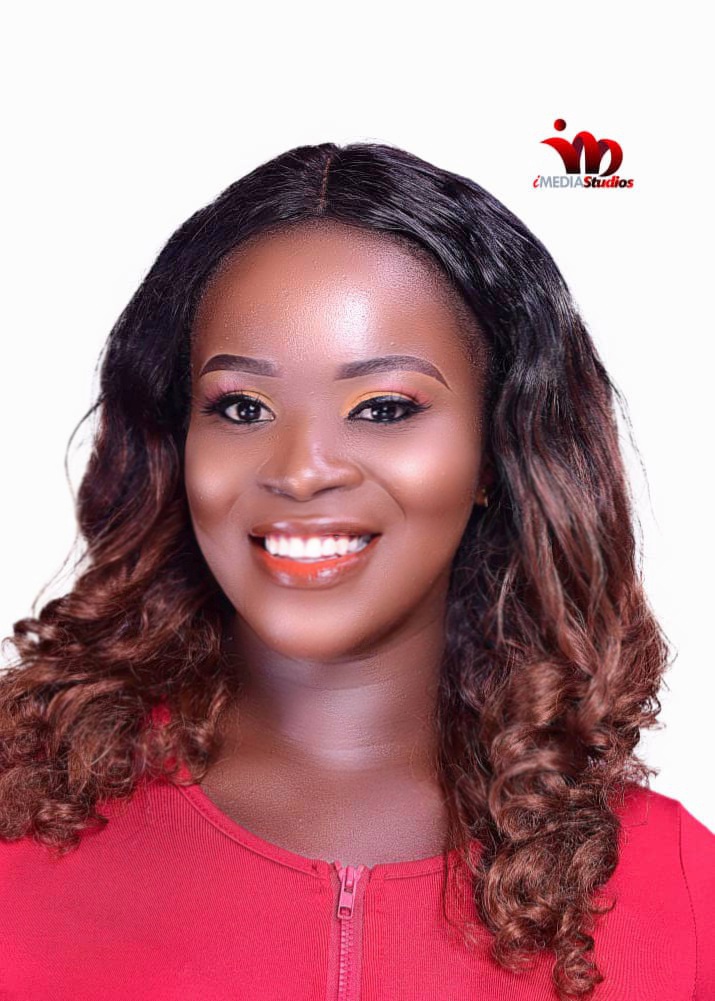
Comments are closed.