In React, it is possible to add attributes or prop or class names to a component only if a certain condition is met.
For example, let’s say you have a general card component with minimal styles :
.card{
width: 300px;
height: 200px;
border: 1px solid black;
}
But then there are certain cases where based on the type prop passed to the component, you want to add a box-shadow to the card with the class below.
.card-shadow{
box-shadow: 5px 10px #888888
}
To do that we’ll have our card component like so:
import React from 'react';
const Card = (props) =>{
return(
<div className={`card ${props.cardType === 'shadow' && "card-shadow" }`}>
{props.children}
</div>
)
}
export default Card;
In the code above, we use template literals to embed the logical AND statement within the className string. The AND operator checks if the cardType prop is equal to shadow and adds the card-shadow class if it is to the card component.
Then in the App component, we can use the Card component to test:
import React from 'react'
import ReactDOM from 'react-dom'
import Card from './Hello'
import './style.css';
class App extends React.Component {
render() {
return (
<div>
<Card cardType="shadow"/>
<Card/>
</div>
)
}
}
ReactDOM.render(
<App />,
document.getElementById('container')
);
Blog Credits: Linda Ikechukwu
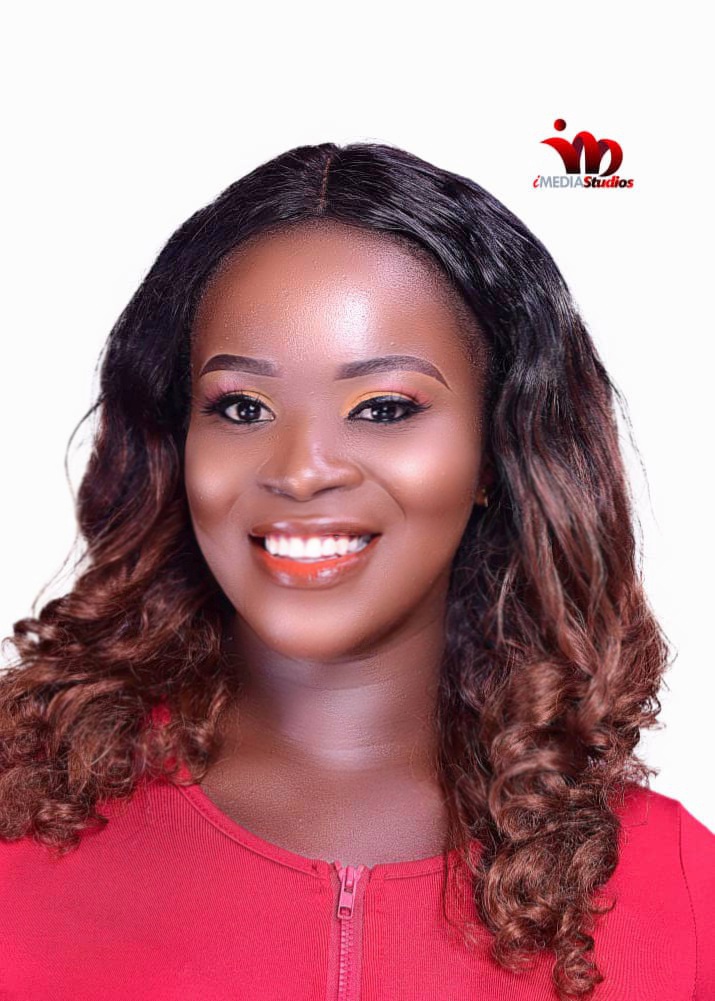
Comments are closed.