When you use methods like getElementsByName or querySelectorAll, to access elements in JavaScript, what is returned is a NodeList.
A NodeList is a collection of HTML DOM nodes representing the HTML elements that met the criteria. At first glance, a NodeList looks like an array, but it is not.
For example, let’s assume that you’re building a synonym word card game. The API you’re using provides an array with objects like this:
{
question: "Synonym of fixed?",
answer: "sorted",
options: ["arrived", "fastened", "sorted", "ran"]
}
The question value is populated into a standalone element, while the values of the options array are populated into a list or collections of divs for the user to click the correct answer. When the user clicks on a choice, we check if the clicked choice corresponds with the value of the answer property. If it doesn’t, we loop through the list and highlight the element containing the correct answer.
Since this snippet isn’t about how to do all of that, we’ll just focus on how to extract that specific list item. There are several ways you can do that and the first is looping through with a for loop.
Using the Nodelist.forEach method and for loop:
const options = document.querySelectorAll('li');
options.forEach(option => {
option.addEventListener('click', () =>{
console.log(option.textContent);
if(option.textContent === "Sorted"){
option.style.color = "green";
}else{
option.style.color = "red";
for(let i = 0; i <= options.length; i++){
if(options[i].textContent === "Sorted"){
options[i].style.color = "green";
}
}
}
})
})
First, we check if the clicked option is the correct one, if it isn’t, we loop through the entire list using a for loop to find the correct one.
Another approach would be to convert the NodeList into an array using array.from. If you want to select an element at a particular position, then you can use the NodeList.item method.
Blog Credits: Linda Ikechukwu
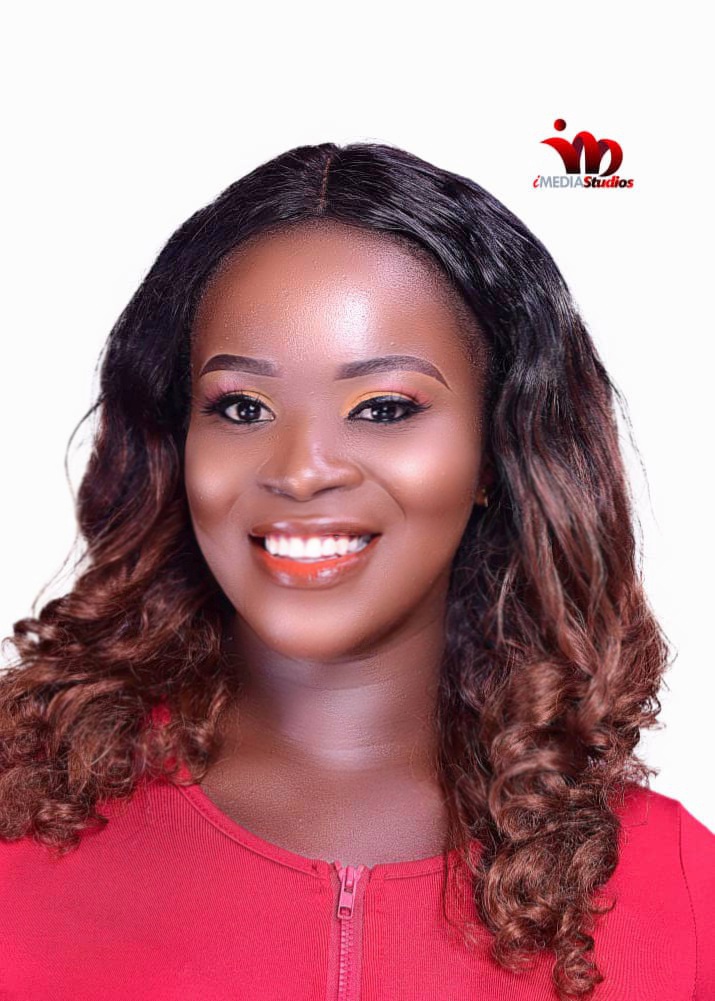
Comments are closed.