Sometimes if you’re working on password inputs, you may want to be able to hint to your users when their CAPS-lock key is on, so they don’t make mistakes.
JavaScript provides a KeyboardEvent event object that can be used to determine the state of various keys, like caps lock, on modern browsers and IE9+.
The KeyboardEvent object has a getModifierState() method. The getModifierState method returns the current state of the modifier key specified: If the modifier is active (that is, the modifier key is pressed or locked), it returns true; otherwise, it returns false.
First, let’s create our input:
<form>
<input type="password" id="password" placeholder="Enter password">
<span id="hint"></span>
</form>
Then let’s get into JavaScript:
const passwordInput = document.querySelector('#password');
const passwordHint = document.querySelector('#hint');
passwordInput.addEventListener('keydown', (e) => {
passwordHint.textContent = e.getModifierState('CapsLock')?'Hint: Your caps lock is on' : '';
});
In the code above, we select both input and hint span with querySelector, then, we add an eventlistener
to the input for every keydown event, to check if the caps lock is on. So, every time the user types in a character, it will check if the caps lock is on and show the hint.
Blog Credits: Linda Ikechukwu
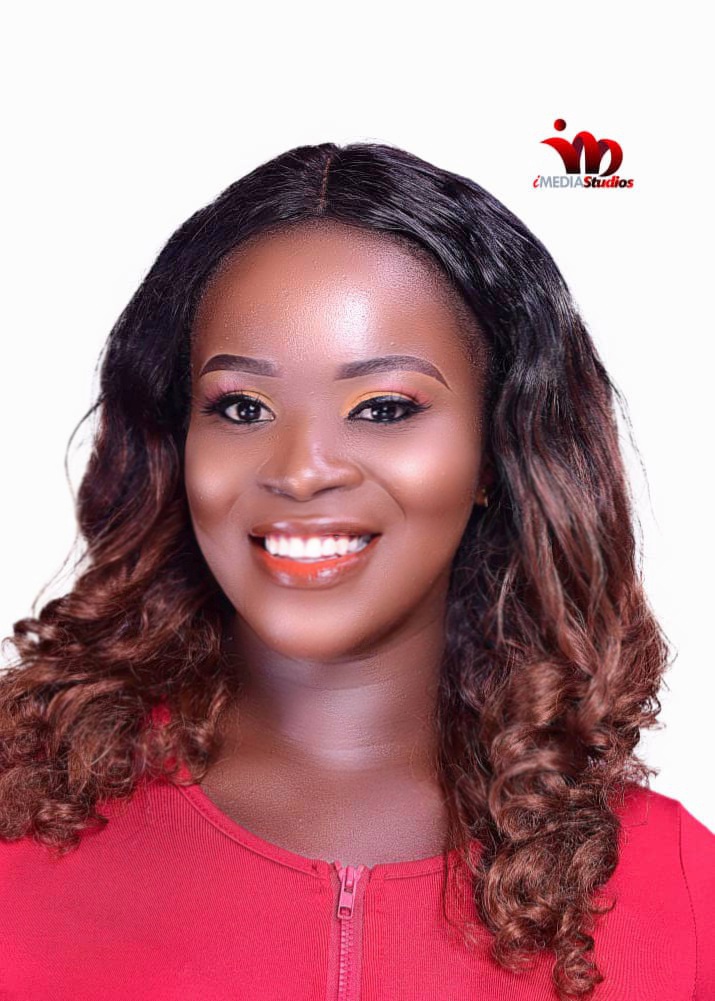
Comments are closed.