In HTML, the focus state highlights which element is currently in view and can receive keyboard events at any particular point. For most web users, the keyboard is their means of navigating through web pages, either due to a disability or preference. Whichever the case may be, having a good focus strategy will make it easier and quicker for more users to navigate your site, which in turn makes them more productive.
There are certain cases where you may want an input focused as soon as it is rendered because you want the user to start typing immediately, without using the mouse or tabbing to navigate to it.
Here are the different ways to do that:
1. Using autoFocus
The React autoFocus
props are modeled after the HTML autofocus property, which sets focus on the element it is attached to as soon as the page containing that element loads.
The autoFocus
prop is useful for cases where such input or the form containing the input is the main attraction of the page, like the Google search homepage or a sign-up/sign-in page.
import React, { Component } from "react";
const PageWithForm = () =>{
return (
<div>
<input
type="text"
placeholder="Type something here"
autoFocus
/>
</div>
);
}
export default PageWithForm;
Note: There may be some weird cases in which the autoFocus prop will not work. For example, if you render the React component into a detached element. In such cases, you can use the focus method below.
2. Using focus()
The focus method is a side effect in React because it is a native HTML API that needs to be called on the HTML DOM and not the virtual DOM. To do that, we need to use refs.
This method is great for cases where you need to set focus on an input conditionally.
For example, when a button is clicked to display a ‘subscribe to our newsletter’ modal containing an email input:
import React, { useState, useRef} from "react";
const PageWithForm = () => {
const inputElement = useRef(null);
const [showModal, setShowModal] = useState(false);
const displayModal = () =>{
setShowModal(true);
setTimeout(()=>{inputElement.current && inputElement.current.focus()}, 1);
}
return (
<div>
<button onClick={displayModal}>Subscribe</button>
{showModal &&
<div classs="modal">
<input
type="text"
placeholder="Type something here"
ref={inputElement}
/>
</div>
}
</div>
);
};
export default PageWithForm;
We used setTimeout
in the displayModal
function to wait until the input is properly visible after the setShowModal
call, before calling focus on it. Else, it won’t work.
Note: You can also use the focus
method to set focus on an input just after the page is mounted, like in the first example using the useEffect
hook:
useEffect(() => {
if (inputElement.current) {
inputElement.current.focus();
}
}, []);
Blog Credits: Linda Ikechukwu
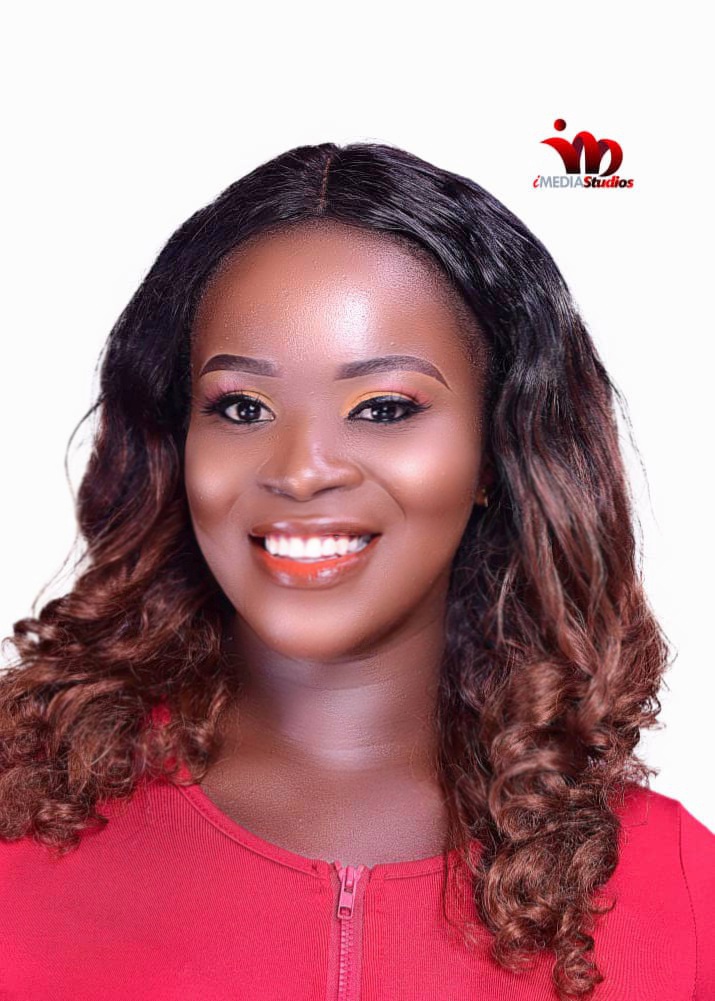
Comments are closed.