Introduction
In the Java programming language, it is not a real challenge to work with a limited number of threads, plus it is fairly easy to manage the execution of one or two threads simultaneously. However, it can become increasingly difficult to handle a substantial number of threads. That is where the executor can help with the creation and management of simultaneous threads.
The Java ExecutorService interface provides management for running many tasks concurrently.
What is an executor?
An executor can use runnable classes without the need for creating a new thread through the reuse of an existing thread. Handling multiple threads is a feature of the Executor Framework.
There are 3 components included:
- Executor Framework
- Thread Pools
- Fork/Join
Embold usage
Why does Embold use Executor?
- Embold processes multiple files parallelly in a few seconds.
- Multiple processes running simultaneously are handled by an executor. For example, the scanning of multiple languages happens in parallel.
- Data accuracy and inter-thread process communication are important. These processes are interdependent. For example, when parsing is complete the calculation of rating is started automatically.
Embold uses FixedThreadPool which is normally configurable using the config file.
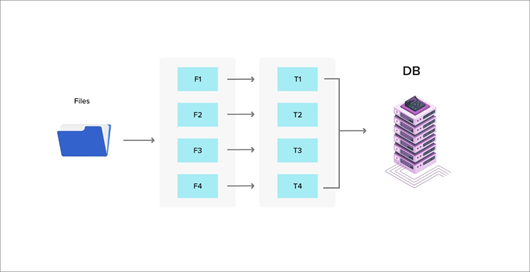
Possible Thread Leak In Executor Service is a unique Java custom code check that helps detect unwanted shutting down of the Executor service.
Rule Name: Possible Thread Leak In Executor Service
Description: Thread leak can be possible if ExecutorService is not getting shut down. There is a queue of tasks between client threads and the thread pool. When the normal thread finishes the “run” method (Runnable or Callable), it will be passed to the garbage collector for collection. But with ExecuterService, threads will be simply put on hold and they will not be selected for garbage collection. Therefore, the shutdown is needed for ExecutorService.
KPI: Resource Utilization
Example:
Non-Compliant code
class Demo {
// ExecutorService is not closed anywhere
public void process1() {
ExecutorService executorService = Executors.newSingleThreadExecutor();
executorService.execute(new Runnable() {
@Override
public void run() {
try {
doTask();
} catch (Exception e) {
logger.error("indexing failed", e);
}
}
});
}
}
Compliant code:
class Demo {
public void process2() {
ExecutorService executorService = Executors.newSingleThreadExecutor();
executorService.execute(new Runnable() {
@Override
public void run() {
try {
doTask();
} catch (Exception e) {
logger.error("indexing failed", e);
}
}
});
executorService.shutdown();
}
}
Summary
In general, the ExecutorService will not automatically shut down while there are any tasks in the queue. One should confirm the count of threads in a pool to avoid the unnecessary overhead for this ExecutorService. It can help to run the application tasks efficiently.
Developers can leverage using this Execution Framework to reduce the complexities involved in executing multiple threads.
Comments are closed.