Event listeners in JavaScript are used to act in response to user events. User events are any interactive activities that a user does while on your website. It can be anything like clicking a button, typing into an input field, pressing the keyboard, etc. So, you could use the event listener to monitor when a user mouses over a certain portion of your website to maybe show them a modal.
In JavaScript, event listening is done with the DOM API’s addEventListener()
method. The syntax for the addEventListener
method is as follows:
element.addEventListener(event, function)
‘element’ represents the HTML element on which you want to react to a user event. ‘event’ is a string that represents which user activity you’re listening for (e.g click, mouseover, etc). See here for a complete list of JavaScript events. And lastly, ‘function’ is a function that contains the action that will be run in response to the event on the specified element.
So, if we want to show content at the click of a button, using the addEventListener
would be:
//html
<button class=”red-button”>Click me</button>
//JavaScript
let redButton = document.querySelector('.red-button');
redButton.addEventListener('click', ()=>{
console.log(“You’ve clicked the button”)
})
Blog Credits: Linda Ikechukwu
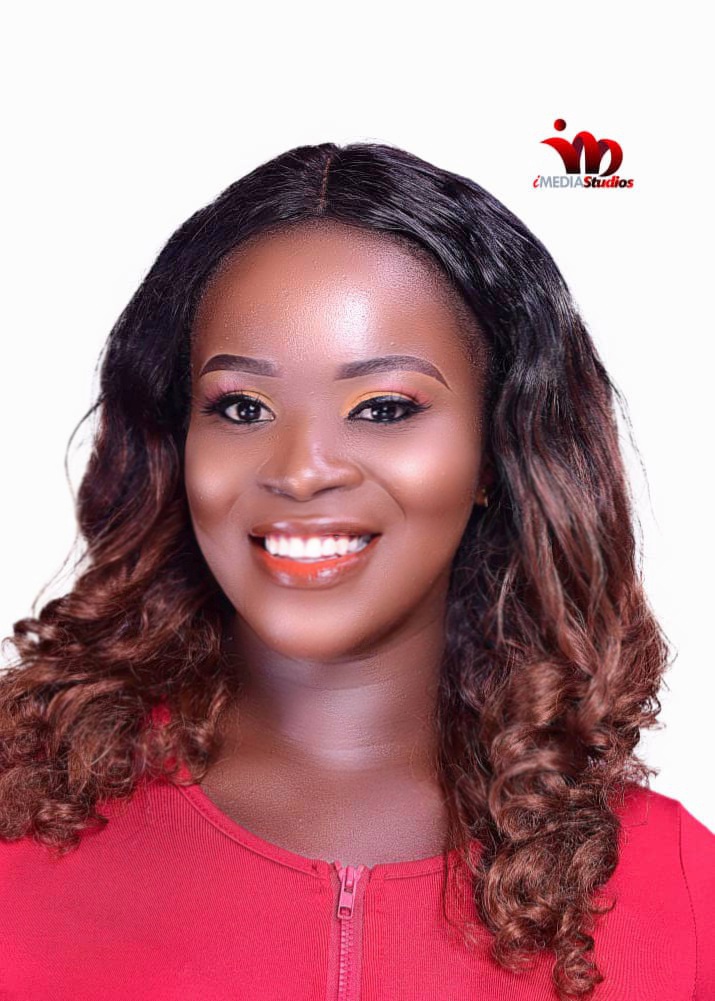
Comments are closed.