There are two three-dot (…) operators in JavaScript: Rest and Spread operator, which was both introduced as part of the ES6 update to the JavaScript language. They’re easily confused with one another because they have the same symbol. But, they’re not the same. The difference is in the context and usage.
The Rest Operator
You can think of the rest operator as a collector. It gathers several values into an array.
It is used to pass an indefinite amount of arguments into a function. This comes in handy when you’re not sure of the number of arguments a function would receive when it is invoked.
For example, assuming you want to write a function that takes in any quantity of numbers and sums it all together with a random number. You have no idea the quantity of numbers the function will receive, so you can use a rest
operator.
const calcTotal = (randomNo, ...numbers) => {
let sumTotal= 0;
for (let number of numbers) {
sumTotal += number
}
console.log(numbers)
return sumTotal + randomNo;
}
calcTotal(5, 10, 13, 7, 8, 17, 89, 10)
//numbers [10, 13, 7, 8, 17, 89, 10]
// Outputs 159
calcTotal (10, 7,9,8,3,24,76,46,54,3,2,69,16, 19)
//numbers [7,9,8,3,24,76,46,54,3,2,69,16, 19]
//Outputs 346
As you can see, the first argument is assigned to the randomNo
parameter while all the other arguments are gathered into the numbers
array using the rest operator.
The Spread Operator
The Spread operator is the exact opposite of the rest operator. You can think of it as a distributor. Unlike the Rest operator, it distributes or unpacks the entries of an iterable entity (strings, arrays, objects, e.t.c) into standalone values.
For example, imagine that you want to pass each number contained in the arr
array into the sum
function below:
const arr = [100, 67, 8]
const sum = (x,y,z) => {
let total = x + y + z;
return total
}
As you can see, the sum
function expects individual arguments, not an array. In this case, you can use the Spread operator to unpack the elements of the arr
array into standalone variables.
sum (...arr)
// Output 175
You can also use the Spread operator to combine the elements of different arrays into one:
const arr1 = [8,10, 6]
const arr2 = [7,9,11]
const mergedArr = [...arr1, ...arr2]
An easier way to distinguish
- When (…) occurs at the end of a function declaration or expression, then it is a Rest operator.
- When (…) occurs in a function invocation or outside a function, then it’s a spread operator.
Blog Credits: Linda Ikechukwu
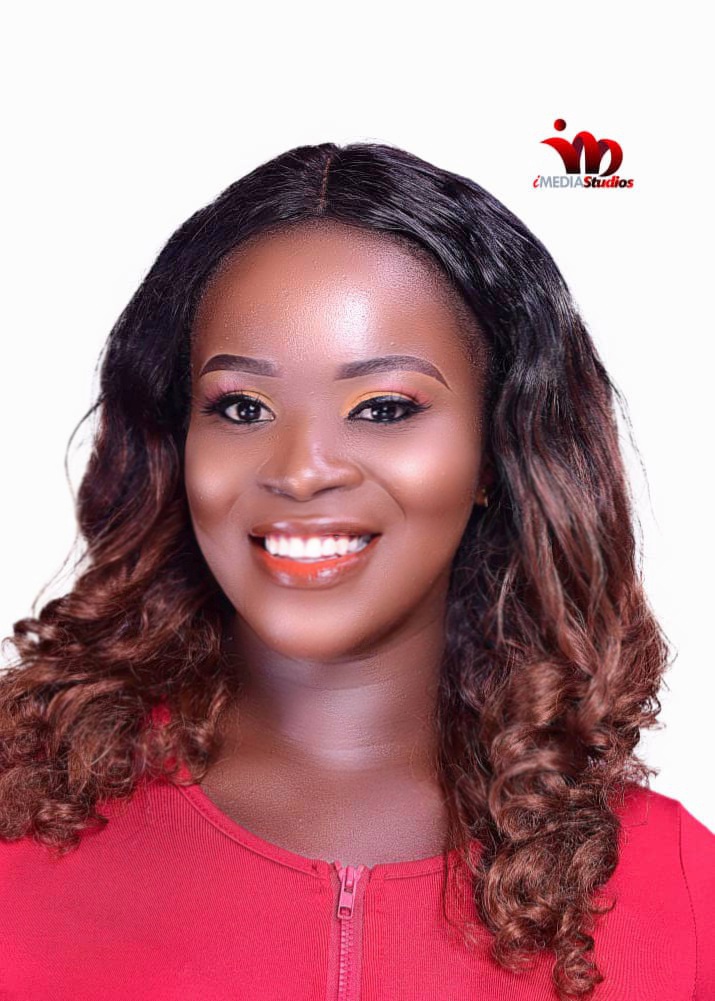
Comments are closed.