The need to format currency according to localization is a common task in JavaScript. Previously, this would have been done with the toLocaleString method, which has several shortcomings. Now, thanks to the ES6 internalization API, it’s become easier to adapt currency representation for audiences from varied locations in JavaScript.
The internalization API made available via the Intl object provides access to the NumberFormat constructor, which enables language-sensitive number formatting.
The syntax is:
new Intl.NumberFormat(locales, options)
Explaining the parameters:
- locales: this is a string that represents language and region settings, and is made up of language code and the country code, e.g en-NG. See the complete list of language codes and country codes.
- options: this is an object which contains two properties; currency (currency to use in currency formatting, e.g USD) and style (the formatting style to use, the currency is the value for currency formatting)
For example, to format currency to number to Nigerian niara, we’ll initialize a new instance of the constructor like so:
const nigerianCurrencyFormat = new Intl.NumberFormat('en-NG', {
currency: 'NG'
style: 'currency',
});
For another country like Canada, we can have:
const canadianCurrencyFormat = new Intl.NumberFormat('en-CAD', {
currency: 'CAD'
style: 'currency',
}).
To use, we’ll call format()
on each:
nigerianCurrencyFormat.format(1000) //₦1,000.00
canadianCurrencyFormat.format(1000) // 'CA$ 100.00'
And that’s it. You can expand this to get the user’s geolocation details, and then based on their country code apply the right conversion and formatting.
Blog Credits: Linda Ikechukwu
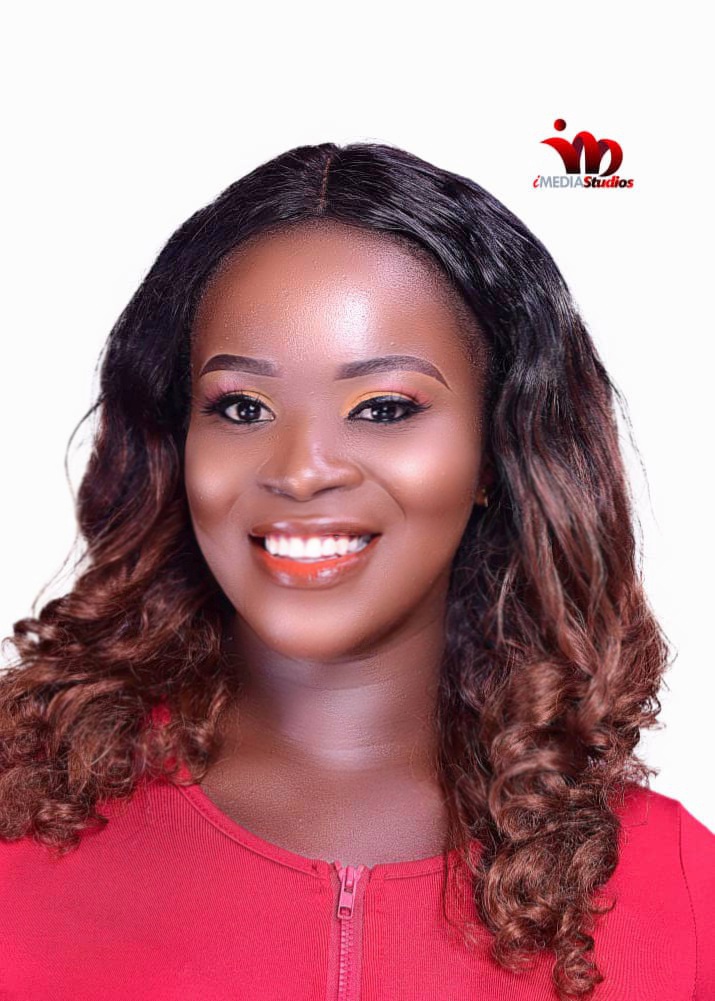
Comments are closed.